bool, complex, float, intbytearray, bytes, strdict, frozenset, list, set, tuplememoryview, object, property, range, slice, type
Draft for Information Only
Content
Python Built-in Class Functions bool() Parameters Remarks complex() Parameters Remarks float() Parameters Remarks int() Parameters Remarks Source and Reference
Python Built-in Class Functions
The Python interpreter has some built-in class functions.
bool()
class bool([𝑥])
Parameters
bool()to return a bool object, True or False
[𝑥]optional, to specify the object to be converted from
Remarks
- The
bool class is a subclass of int
- The possible instances of
bool class are True and False. There is no subclass further.
- If optional 𝑥 is omitted, then
bool() returns False
- 𝑥 can be any object.
- Given 𝑥 is converted using the standard truth testing procedure.
Trueby default, an object is considered true.
Falseclass of object that either a __bool__() method that returns False or a __len__() method that return zero.
constants defined to be false: None and False
zero of any numeric type: 0, 0.0, 0𝑗, Decimal(0), Fraction(0,1), etc.
empty sequences and collections: '', (), [], {}, set(), range(0), etc
complex()
class complex([real[, imag]])
Parameters
complex()to return a complex number.
[real]optional, to specify the object, a string or number, to be converted from
[imag]optional, but real must be given, to specify the imaginary part of a number
Remarks
- Given
real can be specified as following
stringThe given string of parameter real will be interpreted as a complex number. Therefore, the parameter imag should be omitted. Besides, the string must not contain whitespace around the central + or - operator.
numericThe argument of real can be numeric of any type including complex number
omittedthe default is 0
- The argument of
imag can be numeric of any type including complex number. However the argument can never be a string. If parameter imag is omitted, then the default is zero.
- If both arguments are omitted,
complex() returns 0j.
- The
complex() constructor serves as a numeric conversion like int() and float
- For a general Python object 𝑥,
complex(𝑥) delegates to 𝑥.__complex__(). If __complex__() is not defined then it falls back to __float__(). If __float__() is not defined then it falls back to __index__()
float()
class float([𝑥])
Parameters
float()to return a floating point number
[𝑥]optional, to specify the object to be converted from
Remarks
- if argument is omitted, floating point number 0.0 is returned.
- if argument is a string, the string should contain a decimal number, optionally preceded by a sgn, and optionally embedded in whitespace. The optional sign may be
+, -. A + has no effect on the value produced. The argument may also be a string representing a NaN, not-a-number, or a positive or negative infinity. The leading and trailing whitespace characters are removed
sign::="+" | "-"
infinity::="infinity" | "inf"
nan::="nan"
numeric_value::=floatnumber | infinity | nan
numeric_string::=[sign] numeric_value
floatnumber is the form of a Python floating-point literal. Case is not significant.
- if the argument is an integer or a floating point number, a floating point number of the same value within the Python's floating point precision is returned. If the argument is outside the range of a Python float, an
OverflowError will be raised
- For a general Python object 𝑥, float(𝑥) delegates to 𝑥.
__float__(). If __float__() is not defined then it falls back to __index__().
int()
class int([𝑥])
class int(𝑥, base=10)
Parameters
int()to return an integer object
𝑥optional, to specify the object to be returned from
base=10to
Remarks
- If no arguments are given,
int() return 0
- If 𝑥 defines
__int__(), int(𝑥) returns 𝑥.__int__(). If 𝑥 defines __index__(), int(𝑥) returns 𝑥.__index__(). If 𝑥 defines __trunc__(), int(𝑥) returns 𝑥.__trunc__(). For floating point numbers, this truncates towards zero.
- If 𝑥 is not a number or if argument
base is given, then 𝑥 must be a string, bytes, or bytearray instance representing an integer literal in radix base. Optionally, the literal can be preceded by +, or -, with no space in between and surrounded by whitespace.
- A base-𝑛 literal consists of the digits 0 to 𝑛-1, with 𝑎 to 𝑧 (or 𝐴 to 𝑍) having values 10 to 35. The default
base is 10. The allowed values are 0, and 2 to 36. Base-2, base-8, and base-16 literals can be optionally prefixed with 0b/0B, 0o/0O, or 0x/0X, as with integer literals in code. Base 0 means to interpret exactly as a code literal, so that the actual base is 2, 8, 10, 16, and so that int('010',0) is not legal, while int('010') is, as well as int('010',8)
Source and Reference
©sideway
ID: 200601902 Last Updated: 6/19/2020 Revision: 0
|
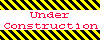 |